这里,statement(s)
可能是一个单一的语句或者是一个具有统一缩进的语句块。condition
可以是任意表达式,而 true
是任何非零值。一旦表达式变为假,程序控制就会传递给紧跟在循环后的下一行。
如果它未能变为假,循环将继续运行,并且不会停止除非强行停止。这样的循环称为无限循环,在计算机程序中是不希望出现的。
while 循环的语法
Python 中 while
循环的语法如下:
while expression:
statement(s)
在 Python 中,所有在编程结构之后由相同数量字符空间缩进的语句都被认为是一个单一的代码块的一部分。Python 使用缩进来作为其分组语句的方法。
while 循环流程图
下面的流程图展示了 while
循环的工作原理:
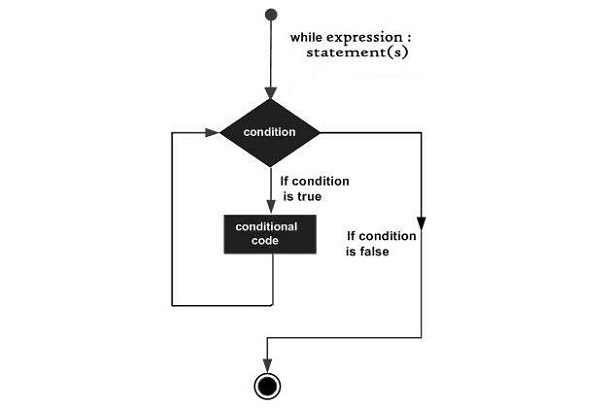
示例 1
下面的例子展示了 while
循环的工作方式。这里,迭代一直进行到 count
的值变成 5。
count = 0
while count < 5:
count += 1
print("Iteration no. {}".format(count))
print("End of while loop")
执行此代码将产生以下输出:
Iteration no. 1
Iteration no. 2
Iteration no. 3
Iteration no. 4
Iteration no. 5
End of while loop
示例 2
这里有一个使用 while
循环的另一个例子。对于每次迭代,程序都会请求用户输入,并且一直重复直到用户输入了一个非数字字符串。isnumeric()
函数在输入为整数时返回真,否则返回假。
var = '0'
while var.isnumeric() == True:
var = input("enter a number..")
if var.isnumeric() == True:
print("Your input", var)
print("End of while loop")
运行此代码将产生以下输出:
enter a number..10
Your input 10
enter a number..100
Your input 100
enter a number..543
Your input 543
enter a number..qwer
End of while loop
Python 无限 while 循环
如果一个条件永远不变成假,则循环变成无限循环。在使用 while
循环时,必须谨慎,因为有可能这个条件永远不会解析为假值。这导致一个永不停止的循环。这样的循环被称为无限循环。
无限循环可能在客户端/服务器编程中有用,其中服务器需要连续运行以便客户端程序可以根据需要与其通信。
示例
让我们通过一个例子来理解 Python 中无限循环是如何工作的:
var = 1
while var == 1:
num = int(input("Enter a number :"))
print("You entered: ", num)
print("Good bye!")
执行此代码将产生以下输出:
Enter a number :20
You entered: 20
Enter a number :29
You entered: 29
Enter a number :3
You entered: 3
Enter a number :11
You entered: 11
Enter a number :22
You entered: 22
Enter a number :
Traceback (most recent call last):
File "examples\test.py", line 5, in
num = int(input("Enter a number :"))
KeyboardInterrupt
上面的例子进入了一个无限循环,并且你需要使用 CTRL+C 来退出程序。
Python while-else 循环
Python 支持将 else
语句与 while
循环关联。如果 else
语句与 while
循环一起使用,那么当条件变为假之前,控制转移到主执行线时,将执行 else
语句。
带有 else 语句的 while 循环流程图
下面的流程图展示了如何使用 else
语句与 while
循环:
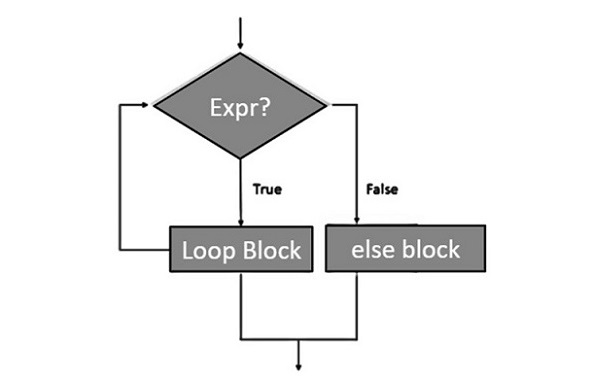
示例
下面的例子展示了 else
语句与 while
语句的组合使用。只要 count
小于 5,就打印迭代次数。当它变成 5 时,在 else
块中的打印语句被执行,然后控制权传递给主程序中的下一个语句。
count = 0
while count < 5:
count += 1
print("Iteration no. {}".format(count))
else:
print("While loop over. Now in else block")
print("End of while loop")
运行上述代码将打印以下输出:
Iteration no. 1
Iteration no. 2
Iteration no. 3
Iteration no. 4
Iteration no. 5
While loop over. Now in else block
End of while loop
单个语句套件
类似于 if
语句的语法,如果你的 while
子句只包含一个单一的语句,它可以放在与 while
头部相同的行上。
示例
下面的例子展示了如何使用单行 while
子句。
flag = 0
while (flag): print("Given flag is really true!")
print("Good bye!")
当你运行这段代码时,它将显示以下输出:
Good bye!
将 flag
值更改为 "1" 并尝试上述程序。如果你这样做,它将进入无限循环,并且你需要按下 CTRL+C 键来退出。