do-while
循环类似于 while
循环,不同之处在于 do-while
循环至少保证执行一次。这是因为 do-while
循环是一个出口控制循环,其中条件是在执行完循环体之后才进行检查的。
do-while
循环的语法
do {
} while (布尔表达式);
do-while
循环的执行过程
注意,布尔表达式出现在循环的末尾,因此在测试布尔表达式之前,循环中的语句至少执行一次。
如果布尔表达式为真,控制权将跳回到 do
语句,循环体内的语句将再次执行。这个过程将重复进行,直到布尔表达式为假。
流程图
下图显示了 Java 中 do-while
循环的流程图(执行过程)。
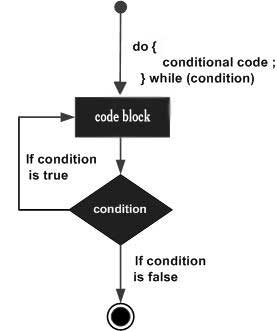
do-while
循环示例
示例 1:使用 do-while
循环打印一个范围内的数字
在这个例子中,我们展示了如何使用 do-while
循环来打印从 10 到 19 的数字。我们初始化了一个 int
类型的变量 x
为 10。然后在 do-while
循环中,我们在循环体内部打印 x
的值并将 x
的值增加 1。在循环体外部,我们检查 x
是否小于 20。do-while
循环将持续运行直到 x
变为 20。一旦 x
达到 20,循环将停止执行,程序退出。
public class Test {
public static void main(String args[]) {
int x = 10;
do {
System.out.print("value of x : " + x );
x++;
System.out.print("\n");
} while (x < 20);
}
}
输出
value of x : 10
value of x : 11
value of x : 12
value of x : 13
value of x : 14
value of x : 15
value of x : 16
value of x : 17
value of x : 18
value of x : 19
示例 2:使用 do-while
循环打印数组元素
在这个例子中,我们展示了如何使用 do-while
循环来打印数组的内容。我们创建了一个名为 numbers
的整数数组,并初始化了一些值。我们在循环中创建了一个名为 index
的变量来表示数组的索引,并检查它是否小于数组的大小。在 do-while
循环体内,我们使用索引来打印数组的元素。index
变量每次增加 1,直到 index
达到数组的大小,循环退出。
public class Test {
public static void main(String args[]) {
int [] numbers = {10, 20, 30, 40, 50};
int index = 0;
do {
System.out.print("value of item : " + numbers[index] );
index++;
System.out.print("\n");
} while (index < 5);
}
}
输出
value of item : 10
value of item : 20
value of item : 30
value of item : 40
value of item : 50
示例 3:实现无限 do-while
循环
可以通过将 "true" 作为条件表达式来实现无限的 do-while
循环。
public class Test {
public static void main(String args[]) {
int x = 10;
do {
System.out.print("value of x : " + x );
x++;
System.out.print("\n");
} while (true);
}
}
输出
value of x : 10
value of x : 11
value of x : 12
value of x : 13
value of x : 14
...
ctrl+c
请注意,这个无限循环将一直运行,直到你手动中断程序(例如,按下 Ctrl+C
)。在实际编程实践中,你应该谨慎使用无限循环,因为它们可能导致程序挂起。