队列接口是在 java.util
包中提供的,并且它实现了 Collection
接口。队列遵循先进先出(FIFO)的原则,这意味着最先加入的元素也是最先被删除的元素。通常,队列用于在处理之前保存元素。一旦元素被处理,就会从队列中移除,并选择下一个元素进行处理。
队列接口声明
下面是队列接口的声明:
public interface Queue<E>
extends Collection<E>
队列接口方法
以下是队列接口的重要方法,所有实现队列接口的类都需要实现这些方法:
序号 |
方法 & 描述 |
1 |
boolean add(E e) 此方法会在不违反容量限制的情况下立即插入指定元素,并在成功时返回 true ;如果没有可用空间则抛出 IllegalStateException 。 |
2 |
E element() 此方法检索队列头部的元素,但不会将其移除。 |
3 |
boolean offer(E e) 此方法会在不违反容量限制的情况下立即插入指定元素。 |
4 |
E peek() 此方法检索队列头部的元素,但不会将其移除;如果队列为空则返回 null 。 |
5 |
E poll() 此方法检索并移除队列头部的元素;如果队列为空则返回 null 。 |
6 |
E remove() 此方法检索并移除队列头部的元素。 |
继承的方法
这个接口继承了以下接口中的方法:
实现队列的类
以下是一些实现了队列以便使用队列功能的类:
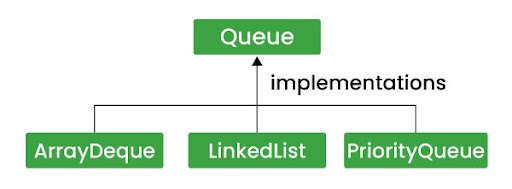
扩展队列的接口
以下是一些扩展队列接口的接口:

队列接口示例
在这个例子中,我们使用 LinkedList
实例来展示队列的添加、查看头部元素以及获取队列大小的操作。
package com.tutorialspoint;
import java.util.LinkedList;
import java.util.Queue;
public class QueueDemo {
public static void main(String[] args) {
Queue<Integer> q = new LinkedList<>();
q.add(6);
q.add(1);
q.add(8);
q.add(4);
q.add(7);
System.out.println("The queue is: " + q);
int num1 = q.remove();
System.out.println("The element deleted from the head is: " + num1);
System.out.println("The queue after deletion is: " + q);
int head = q.peek();
System.out.println("The head of the queue is: " + head);
int size = q.size();
System.out.println("The size of the queue is: " + size);
}
}
输出:
The queue is: [6, 1, 8, 4, 7]
The element deleted from the head is: 6
The queue after deletion is: [1, 8, 4, 7]
The head of the queue is: 1
The size of the queue is: 4